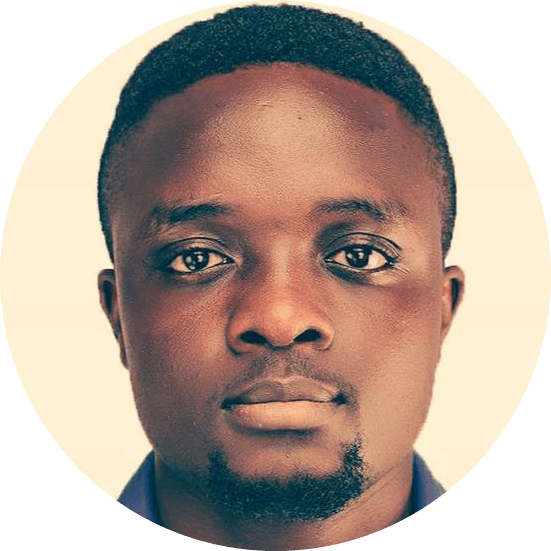
~ min read
Understanding Props in React: D.R.U Technique
A few years ago, when I was first introduced to the concept of Props in React, it seemed strange and difficult to grasp. It took quite a while and a lot of practice to really understand what it is and how it works. I came up with an acronym (DRU) that can help you easily understand props in React.
What are Props?
Props stands for properties and is used to passing data from a parent component to a child component in the Component tree. React has a downward flow of data and this data is transferred using props.
Props are very similar to HTML attributes, such as className, onClick, type, etc for the button element.
D.R.U Technique
This is an acronym for:
-
D: Define prop value in parent component
-
R: Receive prop in child component
-
U: Use prop in child component
When building React applications, the components follow a tree-like structure and data flows from top to bottom. This data is passed down via props from component to component.
The DRU technique provides a structure to easily understand each step needed to pass down and use props within components.
An Example
import React from 'react'
// ParentComponent
function App() {
const productName = 'Car' // D: Define prop value
// R: Receive prop
return <Product name={productName} />
}
// ChildComponent
function Product({ name }) {
// U: Use Prop
return <h1>This is a {name}</h1>
}
As seen above, we first Define the prop value (productName) in the parent component, then we Receive the prop, using the any name of choice, in this case 'name' and then we Use the prop, 'name' in the Product component.
React's "children" Prop
This is a special prop in React. When you nest content between the opening and the closing tag of an element or component, the parent component receives that content in a prop called children
.
In the example above, Product
is a child of App
component.
You can think of a component with a children prop as having a “hole” that can be “filled in” by its parent components with arbitrary JSX. You will often use the children prop for visual wrappers: containers, panels, grids, etc.
Tip
You can specify the default value of a prop when destructuring by putting =
and the default value right after the parameter. This will be the fallback value if no value is specified.
function Product({ name = 'Bicycle' }) {
return <h1>This is a {name}</h1>
}
If no value is passed to the name prop, Bicycle will be used.
Conclusion
This article has explained how props work in React. The DRU technique is breakdown and will help you in your understanding or Props. Good Luck!