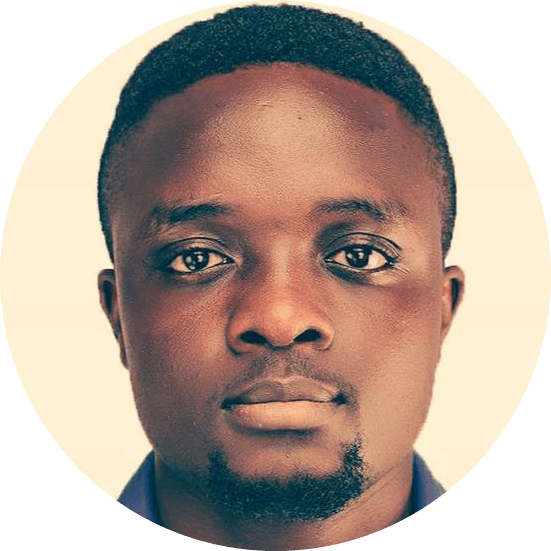
~ min read
The JavaScript you need to know for React
This is a common question among beginners who are willing to learn React JS. While it's a subjective topic, I’ll share my thoughts based on the approach I took when I started and what I would recommend from experience.
Many developers, including myself, choose a "learn as you go" approach when learning React. While this method can seem convenient, it often affects productivity in the long run and may result in knowledge gaps, especially in JavaScript.
There are alot of concepts that would be great to know, however I will focus on the key concepts and features that are important to understand before diving into React.
JavaScript Basics
It's important to have a solid grasp of some fundamental JavaScript concepts, understanding not just how they work but also why they work the way they do. Many features you’ll encounter in React are in JavaScript but are used in slightly different ways. Therefore, a strong understanding of these concepts will make it easier for you to work with them effectively in React.
Some of the basics you need to learn are:
Callback functions
Callbacks are functions are functions passed into another function as an argument that can be used for some kind of action at a later time. For example
function sumValues(num1, num2, callback) {
const sum = num1 + num2
callback(sum)
}
function logSum(sum) {
console.log('THE SUM IS: ' sum)
}
sumValues(1,3, logSum)
// RESULT IN CONSOLE
// THE SUM IS: 4
Read more here
Variable Scope
In JavaScript, this refers to the environment or context in which variables are declared and can be accessed. A solid understanding of this is crucial because it influences how you structure your code, directly affecting how your code behaves and interacts with other parts of your application.
function counter() {
let count = 0 // Private variable
return function () {
count++ // Increment the private variable
console.log(count)
}
}
const increment = counter()
increment() // Output: 1
increment() // Output: 2
increment() // Output: 3
Learn more about scoping here
Array Methods
Array methods are powerful and have been used in almost all applications I have worked on. The following are the most frequently used:
- map
- filter
- find
- includes
- reduce
- some
- every
Here is an example:
const people = [
{
name: 'James',
age: 20,
siblings: [],
},
{
name: 'Thomas',
age: 22,
siblings: ['Louis'],
},
{
name: 'Aseda',
age: 19,
siblings: ['Anthony'],
},
{
name: 'Lucy',
age: 15,
siblings: [],
},
{
name: 'Thompson',
age: 32,
siblings: ['Grace', 'Philip'],
},
]
people.map((person) => person.age)
// [ 20, 22, 19, 15, 32 ]
people.filter((person) => person.age < 20)
// [{name: 'Aseda',age: 19}, {name: 'Lucy', age: 15}]
people.find((person) => person.age === 15)
// { name: 'Lucy', age: 15 }
people.reduce((accumulator, person, ind) => {
// Sum of all siblings
if (person.siblings) {
return accumulator + person.siblings.length
}
return accumulator
}, 0)
// 4
people.some((person) => person.siblings?.includes('Grace'))
// true
people.every((person) => person.siblings?.includes('Grace'))
// false
Learn more about Arrays here
Template Literals
They are sometimes referred to as Template strings and are mostly used for string interpolation and multi-line strings.
const firstName = 'Neville'
const lastName = 'Kati'
// Without Template Literals
console.log('My name is ' + firstName + ' ' + lastName + '.')
// My name is Neville Kati.
// With Template Literals
console.log(`My name is ${firstName} ${lastName} .`)
// My name is Neville Kati.
Learn more about Arrays here
Rest and Spread operators
These oparators have are defined with the "..." syntax and could be thought of as an operation on a collection of values. It could take different meanings at different contexts, hence learning its nuances will help you.
- The Rest Operator allows a function to accept an indefinite number of arguments as an array
function add(...input) {
let sum = 0
for (let i of input) {
sum += i
}
return sum
}
console.log(add(1, 2)) //3
console.log(add(1, 2, 3)) //6
console.log(add(1, 2, 3, 4, 5)) //15
Learn more about Rest Operators here
- The Spread Operator is mostly used when you want to duplicate the content of an array or object.
const firstArray = [5, 10, 15, 20, 25, 30]
const newArray = [...firstArray, 35, 40]
console.log(newArray) // [5, 10, 15, 20, 25, 30, 35, 40]
Learn more about Spread Operators here
Nullish coalescing operator '??'
This is quite handy when you have "null"
or "undefined"
values and need to provide a fallback value.
const result = a !== null && a !== undefined ? a : b
// Here is one way of assigning a value to the variable result
// But this is combasom and a little hard to Read
// This can be simplified to:
const result = a ?? b
// This just checks if a is neither "null" nor "undefined" then return a else return b
Learn more about Nullish coalescing oparators here
Parameter defaults
This is a way of assigning default values to function parameters.
// This function receives parameters a and b
// b here has been assigned a default value 0
const add = (a, b = 0) => a + b
// The above function can be rewritten as
function add(a, b) {
b = b === undefined ? 0 : b
return a + b
}
Ternary Operators
It is used to conditionally display data. It can be looked at as a way of writing if ... else
statements in JSX.
const filledUp = false // or true
const message = filledUp
? 'The bottle has soda!'
: 'The bottle may not have soda '
// is the same as
let message
if (filledUp) {
message = 'The bottle has soda!'
} else {
message = 'The bottle may not have soda :-('
}
// in React:
function Drinks() {
const drinkAlternatives = [
{
name: 'Coca Cola',
quantity: 4,
},
{
name: 'Fanta',
quantity: 2,
},
]
return (
<>
{drinkAlternatives.length ? (
<ul>
{drinkAlternatives.map((drink) => (
<li key={drink.name}>
<span>
{drink.name} is {drink.quantity} in number
</span>
</li>
))}
</ul>
) : (
<div>There are no drinks. The sadness.</div>
)}
</>
)
}
Learn more about Ternary oparators here
Optional Chaining
This allows you to safely access properties and call functions that may or may not exist.
// this was before optional Chaining
const address = user && user.address && user.address.name
// this is the same with optional chaining
const address = user?.address?.name
// this will run even if "user" is "undefined"
Learn more about Optional chaining here
Destructuring
This is an amazing JavaScript feature; it provides an easy way of accessing values from arrays, or properties of objects into distinct variables.
const names = ['John', 'Carine', 'Anatelle', 'Francis', 'Joy']
const food = {
name: 'Potato',
nutrient: 'Carbohydrates',
cost: '$1.5',
}
// without destructuring, we could access the values of the array and object in this manner
return (
<div>
{food.name} is a made of mainly {food.nutrient}.{names[0]} and {names[1]}{' '}
are not destructured.
</div>
)
// Destructuring provides are much cleaner syntaxt:
const { name, nutrient } = food
const [name1, name2] = names
return (
<div>
{name} is a made of mainly {nutrient}.{name1} and {name2} are destructured
</div>
)
This is such a powerful tool. You can learn more about destructuring here
Promises
This is a huge topic and would probably take some time of practice to get really good at it. Promises help you manage asynchronous operations. A typical one is in making http request using fetch()
Async/await is a special syntax for dealing with promises. The two go hand-in-hand.
// Function using async/await
async function fetchData() {
try {
const result = await new Promise((resolve) => {
// logic for asynchronous task
setTimeout(() => {
resolve('Data fetched successfully!')
}, 2000)
})
console.log(result) // Runs when the promise is resolved
} catch (error) {
console.log(error) // Runs when the promise is rejected/fails
}
}
Learn more here
Learn more about Promises here
Learn more about Async/await here
Conclusion
There are a lot more JavaScript features and concepts that you would need to understand when building React applications, but these are some Key ones in my opinion that I think are really important to know and understand.
I hope you found this useful.
Good Luck!